Example code
by Salim Benhouhou IT Support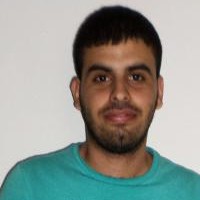
Salim Benhouhou
IT Support
The classic hello world program can be written as follows:
print("Hello World!")
Comments use the following syntax, similar to that of SQL and VHDL:
-- A comment in Lua starts with a double-hyphen and runs to the end of the line.--[[ Multi-line strings & comments
are adorned with double square brackets. ]]
The factorial is an example of a recursive function:
function factorial(n) if n == 0 then return 1 else return n * factorial(n - 1) endend
The second form of the factorial function originates from Lua's short-circuit evaluation of boolean operators, in which Lua will return the value of the last operand evaluated in an expression:
function factorial2(n) return n == 0 and 1 or n * factorial2(n - 1)end
Lua’s treatment of functions as first-class values is shown in the following example, where the print function’s behavior is modified:
do local oldprint = print -- Store current print function as oldprint function print(s) -- Redefine print function if s == "foo" then oldprint("bar") else oldprint(s) end endend
Any future calls to print will now be routed through the new function, and thanks to Lua’s lexical scoping, the old print function will only be accessible by the new, modified print.
Lua also supports closures, as demonstrated below:
function addto(x) -- Return a new function that adds x to the argument return function(y) -- When we refer to the variable x, which is outside of the current -- scope and whose lifetime is longer than that of this anonymous -- function, Lua creates a closure. return x + y endendfourplus = addto(4)print(fourplus(3)) -- Prints 7
A new closure for the variable x is created every time addto is called, so that the anonymous function returned will always access its own x parameter. The closure is managed by Lua’s garbage collector, just like any other object.
Extensible semantics is a key feature of Lua, and the metatable
concept allows Lua’s tables to be customized in powerful and unique
ways. The following example demonstrates an “infinite” table. For any n, fibs[n] will give the nth Fibonacci number using dynamic programming and memoization.
fibs = { 1, 1 } -- Initial values for fibs[1] and fibs[2].setmetatable(fibs, { -- Give fibs some magic behavior. __index = function(name, n) -- Call this function if fibs[n] does not exist. name[n] = name[n - 1] + name[n - 2] -- Calculate and memorize fibs[n]. return name[n] end})
Tables
Tables are the most important data structure (and, by design, the only built-in composite data type) in Lua, and are the foundation of all user-created types.
A table is a collection of key and data pairs, where the data is referenced by key; in other words, it's a hashed heterogeneous associative array. A key (index) can be of any data type except nil. An integer key of 1 is considered distinct from a string key of "1".
Tables are created using the {} constructor syntax:
a_table = {} -- Creates a new, empty table
Tables are always passed by reference:
-- Creates a new table, with one associated entry. The string x mapping to-- the number 10.
a_table = {x = 10}
-- Prints the value associated with the string key, in this case 10.
print(a_table["x"])b_table = a_tableb_table["x"] = 20 -- The value in the table has been changed to 20.print(b_table["x"]) -- Prints 20.
-- Prints 20, because a_table and b_table both refer to the same table.
print(a_table["x"])
As structure
Tables are often used as structures (or objects) by using strings as keys. Because such use is very common, Lua features a special syntax for accessing such fields. Example:
point = { x = 10, y = 20 } -- Create new tableprint(point["x"]) -- Prints 10print(point.x) -- Has exactly the same meaning as line above
As namespace
By using a table to store related functions, it can act as a namespace.
Point = {}Point.new = function (x, y) return {x = x, y = y}endPoint.set_x = function (point, x) point.x = xend
As array
By using a numerical key, the table resembles an array data type.
Lua arrays are 1-based: the first index is 1 rather than 0 as it is for
many other programming languages (though an explicit index of 0 is
allowed).
A simple array of strings:
array = { "a", "b", "c", "d" } -- Indices are assigned automatically.
print(array[2]) -- Prints "b". Automatic indexing in Lua starts at 1.
print(#array) -- Prints 4. # is the length operator for tables and strings.
array[0] = "z" -- Zero is a legal index.
print(#array) -- Still prints 4, as Lua arrays are 1-based.
An array of objects:
function Point(x, y) -- "Point" object constructor return { x = x, y = y } -- Creates and returns a new object (table)endarray = { Point(10, 20), Point(30, 40), Point(50, 60) } -- Creates array of pointsprint(array[2].y) -- Prints 40
Using a hash map to emulate an array normally is slower than using
an actual array; however, Lua tables are optimized for use as arrays to help avoid this issue.
Object-oriented programming
Although Lua does not have a built-in concept of classes, they can be implemented using two language features: first-class functions and tables. By placing functions and related data into a table, an object is formed. Inheritance (both single and multiple) can be implemented via the metatable mechanism, telling the object to lookup nonexistent methods and fields in parent object(s).
There is no such concept as "class" with these techniques, rather prototypes are used as in the programming languages Self or JavaScript. New objects are created either with a factory method (that constructs new objects from scratch) or by cloning an existing object.
Lua provides some syntactic sugar to facilitate object orientation. To declare member functions inside a prototype table, one can use function table:func(args), which is equivalent to function table.func(self, args). Calling class methods also makes use of the colon: object:func(args) is equivalent to object.func(object, args).
Creating a basic vector object:
Vector = {} -- Create a table to hold the class methods
function Vector:new(x, y, z) -- The constructor
local object = { x = x, y = y, z = z }
setmetatable(object, { __index = Vector }) -- Inheritance
return object
end
function Vector:magnitude() -- Another member function
-- Reference the implicit object using self
return math.sqrt(self.x^2 + self.y^2 + self.z^2)
end
vec = Vector:new(0, 1, 0) -- Create a vector
print(vec:magnitude()) -- Call a member function using ":"
print(vec.x) -- Access a member variable using "."
Apr 18th 2011 13:13


The classic hello world program can be written as follows:
print("Hello World!")
Comments use the following syntax, similar to that of SQL and VHDL:
-- A comment in Lua starts with a double-hyphen and runs to the end of the line.--[[ Multi-line strings & comments
are adorned with double square brackets. ]]
The factorial is an example of a recursive function:
function factorial(n) if n == 0 then return 1 else return n * factorial(n - 1) endend
The second form of the factorial function originates from Lua's short-circuit evaluation of boolean operators, in which Lua will return the value of the last operand evaluated in an expression:
function factorial2(n) return n == 0 and 1 or n * factorial2(n - 1)end
Lua’s treatment of functions as first-class values is shown in the following example, where the print function’s behavior is modified:
do local oldprint = print -- Store current print function as oldprint function print(s) -- Redefine print function if s == "foo" then oldprint("bar") else oldprint(s) end endend
Any future calls to print will now be routed through the new function, and thanks to Lua’s lexical scoping, the old print function will only be accessible by the new, modified print.
Lua also supports closures, as demonstrated below:
function addto(x) -- Return a new function that adds x to the argument return function(y) -- When we refer to the variable x, which is outside of the current -- scope and whose lifetime is longer than that of this anonymous -- function, Lua creates a closure. return x + y endendfourplus = addto(4)print(fourplus(3)) -- Prints 7
A new closure for the variable x is created every time addto is called, so that the anonymous function returned will always access its own x parameter. The closure is managed by Lua’s garbage collector, just like any other object.
Extensible semantics is a key feature of Lua, and the metatable
concept allows Lua’s tables to be customized in powerful and unique
ways. The following example demonstrates an “infinite” table. For any n, fibs[n] will give the nth Fibonacci number using dynamic programming and memoization.
fibs = { 1, 1 } -- Initial values for fibs[1] and fibs[2].setmetatable(fibs, { -- Give fibs some magic behavior. __index = function(name, n) -- Call this function if fibs[n] does not exist. name[n] = name[n - 1] + name[n - 2] -- Calculate and memorize fibs[n]. return name[n] end})
Tables
Tables are the most important data structure (and, by design, the only built-in composite data type) in Lua, and are the foundation of all user-created types.
A table is a collection of key and data pairs, where the data is referenced by key; in other words, it's a hashed heterogeneous associative array. A key (index) can be of any data type except nil. An integer key of 1 is considered distinct from a string key of "1".
Tables are created using the {} constructor syntax:
a_table = {} -- Creates a new, empty table
Tables are always passed by reference:
-- Creates a new table, with one associated entry. The string x mapping to-- the number 10.
a_table = {x = 10}
-- Prints the value associated with the string key, in this case 10.
print(a_table["x"])b_table = a_tableb_table["x"] = 20 -- The value in the table has been changed to 20.print(b_table["x"]) -- Prints 20.
-- Prints 20, because a_table and b_table both refer to the same table.
print(a_table["x"])
As structure
Tables are often used as structures (or objects) by using strings as keys. Because such use is very common, Lua features a special syntax for accessing such fields. Example:
point = { x = 10, y = 20 } -- Create new tableprint(point["x"]) -- Prints 10print(point.x) -- Has exactly the same meaning as line above
As namespace
By using a table to store related functions, it can act as a namespace.
Point = {}Point.new = function (x, y) return {x = x, y = y}endPoint.set_x = function (point, x) point.x = xend
As array
By using a numerical key, the table resembles an array data type.
Lua arrays are 1-based: the first index is 1 rather than 0 as it is for
many other programming languages (though an explicit index of 0 is
allowed).
A simple array of strings:
array = { "a", "b", "c", "d" } -- Indices are assigned automatically.
print(array[2]) -- Prints "b". Automatic indexing in Lua starts at 1.
print(#array) -- Prints 4. # is the length operator for tables and strings.
array[0] = "z" -- Zero is a legal index.
print(#array) -- Still prints 4, as Lua arrays are 1-based.
An array of objects:
function Point(x, y) -- "Point" object constructor return { x = x, y = y } -- Creates and returns a new object (table)endarray = { Point(10, 20), Point(30, 40), Point(50, 60) } -- Creates array of pointsprint(array[2].y) -- Prints 40
Using a hash map to emulate an array normally is slower than using
an actual array; however, Lua tables are optimized for use as arrays to help avoid this issue.
Object-oriented programming
Although Lua does not have a built-in concept of classes, they can be implemented using two language features: first-class functions and tables. By placing functions and related data into a table, an object is formed. Inheritance (both single and multiple) can be implemented via the metatable mechanism, telling the object to lookup nonexistent methods and fields in parent object(s).
There is no such concept as "class" with these techniques, rather prototypes are used as in the programming languages Self or JavaScript. New objects are created either with a factory method (that constructs new objects from scratch) or by cloning an existing object.
Lua provides some syntactic sugar to facilitate object orientation. To declare member functions inside a prototype table, one can use function table:func(args), which is equivalent to function table.func(self, args). Calling class methods also makes use of the colon: object:func(args) is equivalent to object.func(object, args).
Creating a basic vector object:
Vector = {} -- Create a table to hold the class methods
function Vector:new(x, y, z) -- The constructor
local object = { x = x, y = y, z = z }
setmetatable(object, { __index = Vector }) -- Inheritance
return object
end
function Vector:magnitude() -- Another member function
-- Reference the implicit object using self
return math.sqrt(self.x^2 + self.y^2 + self.z^2)
end
vec = Vector:new(0, 1, 0) -- Create a vector
print(vec:magnitude()) -- Call a member function using ":"
print(vec.x) -- Access a member variable using "."
Apr 18th 2011 13:13
Sponsor Ads
Comments
No comment, be the first to comment.